Asparagus, the elegant green spears of spring, are more versatile than you might think! This recipe showcases the delicate flavor of asparagus with a simple yet sophisticated preparation, perfect for a weeknight dinner or a special occasion. Whether you’re an experienced cook or a culinary novice, you’ll find this asparagus recipe easy to follow and utterly delightful. Get ready to elevate your dinner game with this amazing asparagus dish!
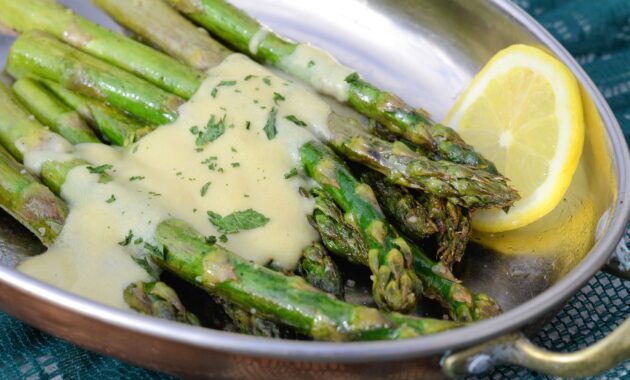
This recipe highlights the natural sweetness of asparagus, enhancing its flavor with a touch of lemon and garlic. It’s quick, it’s easy, and it’s a guaranteed crowd-pleaser! Learn how to cook asparagus to perfection, transforming these spring vegetables into a culinary masterpiece. The best part? This asparagus recipe is incredibly adaptable, allowing you to customize it to your tastes and preferences.
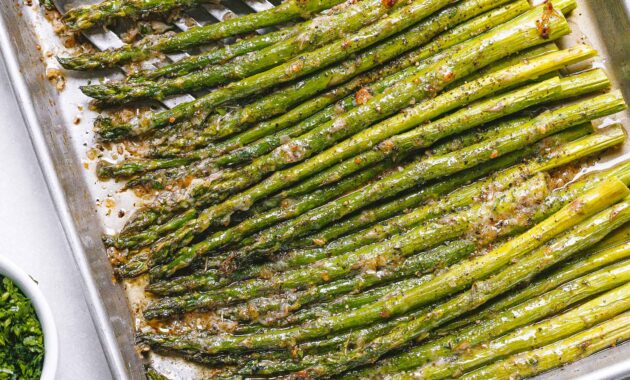
Category | Value |
---|---|
Preparation Time | 10 minutes |
Cooking Time | 15 minutes |
Servings | 4 servings |
Difficulty | Easy |
Nutrition Information (per serving, approximate): Calories: 80, Fat: 2g, Protein: 4g, Carbohydrates: 12g, Fiber: 3g
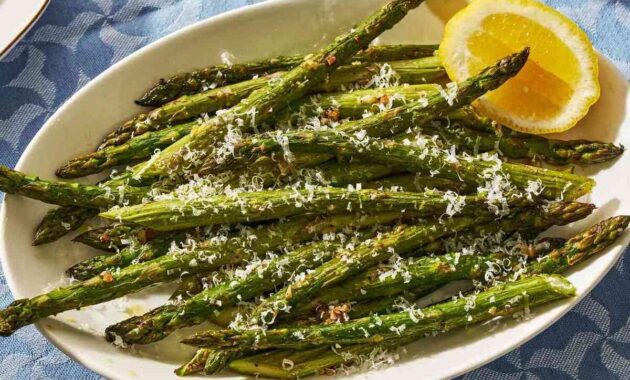
Ingredient | Quantity |
---|---|
Asparagus | 1 pound |
Olive Oil | 2 tablespoons |
Garlic Cloves (minced) | 2-3 |
Lemon Juice | 1 tablespoon |
Salt | To taste |
Black Pepper | To taste |
Parmesan Cheese (optional) | 1/4 cup, grated |
Cooking Instructions
- Wash and trim the asparagus, snapping off the tough ends. For this asparagus recipe, aim for uniformly sized spears for even cooking.
- Heat the olive oil in a large skillet over medium heat. Add the minced garlic and sauté for about 30 seconds, or until fragrant. Be careful not to burn the garlic, as this will impact the flavor of your asparagus.
- Add the asparagus spears to the skillet in a single layer. Avoid overcrowding the pan to ensure even browning and cooking of each asparagus spear.
- Cook the asparagus for 5-7 minutes, turning occasionally, until it’s tender-crisp. The asparagus should be bright green and slightly tender when you pierce it with a fork. This is crucial for the success of this asparagus recipe.
- Remove the asparagus from the heat and stir in the lemon juice, salt, and black pepper. The lemon juice adds a lovely brightness to the asparagus, complementing its natural flavor.
- Serve the asparagus immediately. You can garnish with freshly grated Parmesan cheese if desired. This simple addition elevates the asparagus recipe to new heights.
Serving Suggestions: This simple asparagus recipe is a wonderful side dish for grilled meats, roasted chicken, or fish. It also pairs beautifully with pasta dishes or risotto. The versatility of asparagus shines in this recipe, offering a simple yet delicious accompaniment to countless entrees. For a complete meal, consider pairing your asparagus with some grilled chicken or salmon for a light and healthy dinner. This asparagus recipe complements a variety of proteins, making it a perfect addition to your culinary repertoire.
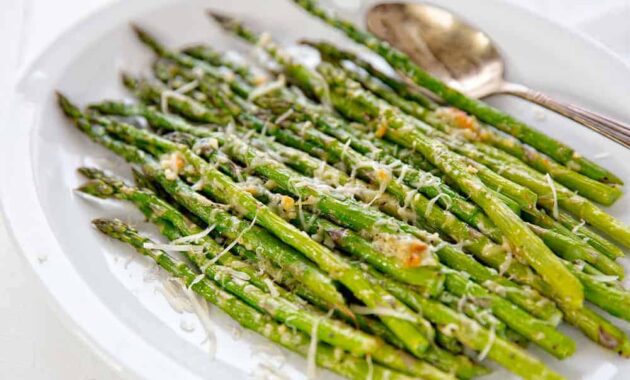
Tips and Notes: For a richer flavor, try adding a tablespoon of butter along with the olive oil. If you prefer a more intense garlic flavor, you can add more minced garlic. Don’t overcook the asparagus! It’s best served tender-crisp, retaining its vibrant green color and slight crunch. This simple asparagus recipe relies on the fresh, vibrant flavor of the asparagus. Properly cooked asparagus adds an exquisite element to any meal, making it a staple among culinary enthusiasts. This easy asparagus recipe is perfect for those short on time, but don’t let its simplicity fool you—it delivers big on taste! Remember to adjust the seasoning to your liking and experiment with different herbs and spices to further enhance the flavor profile of your asparagus. The possibilities are endless with this versatile vegetable! This recipe provides a delightful and accessible way to enjoy the deliciousness of asparagus and provides endless opportunities to customize this asparagus recipe to your preferences.
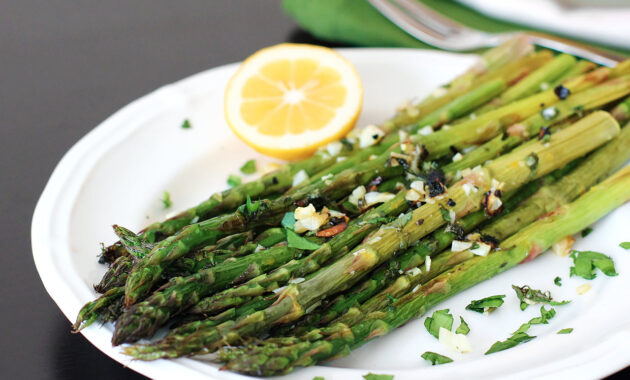